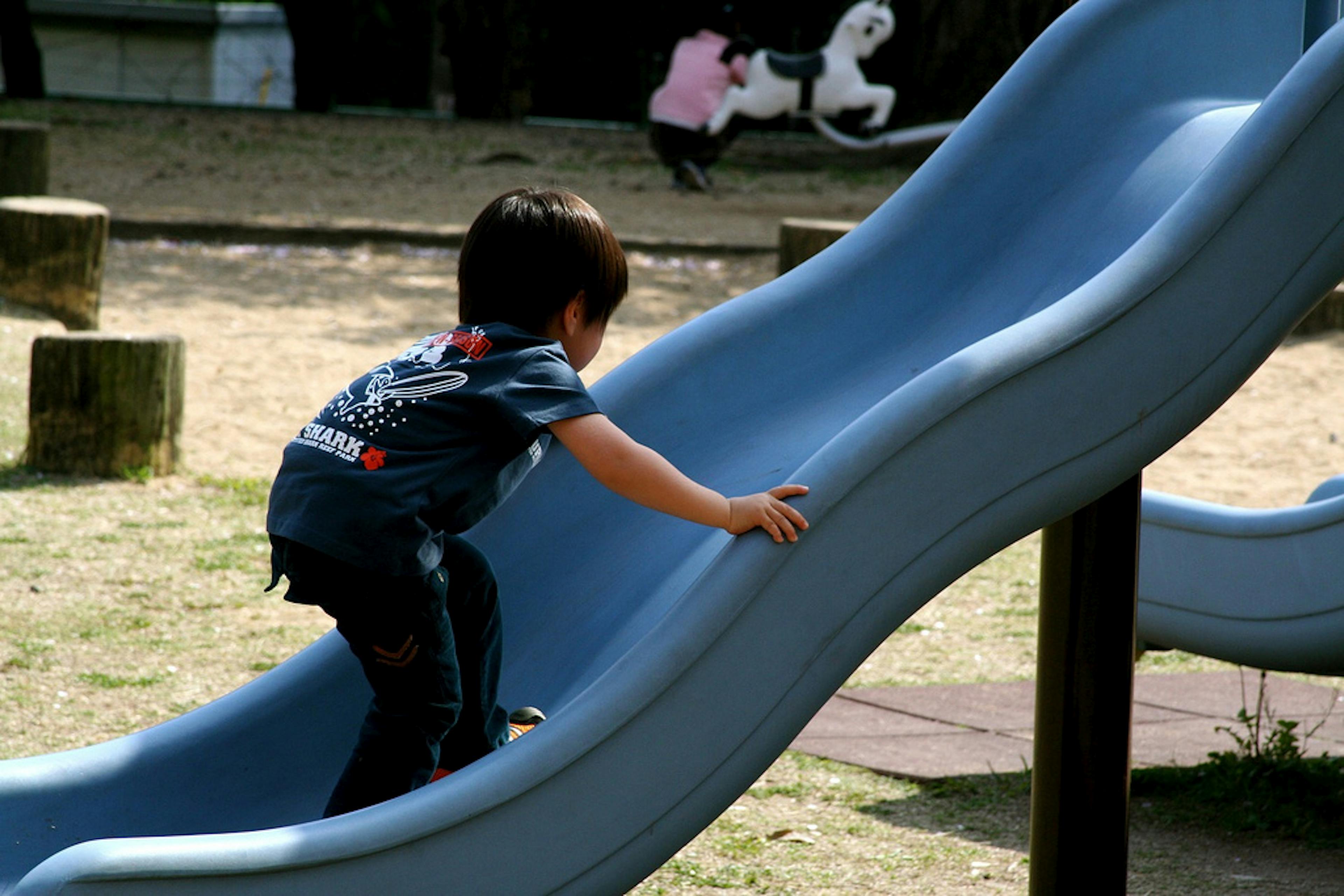
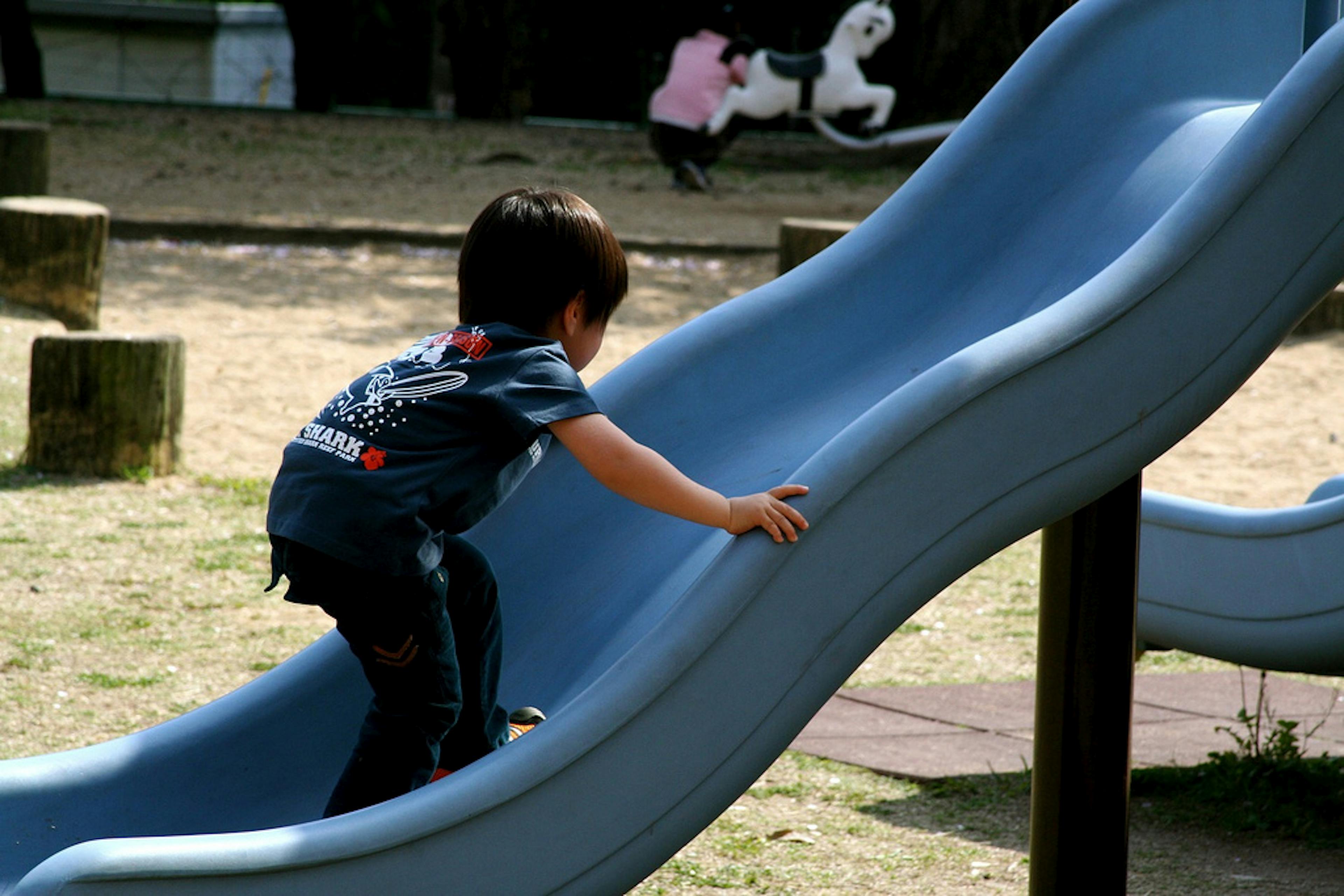
One of the last reasons I still had for using jQuery was the very convenient slideUp(), slideDown(), and slideToggle() functions. Ahh the joy of this satisfying simple transition that if attempted with CSS-only would be near impossible to achieve with the same fluidity and flexibility.
I've at least a few times broke the well-known rule of direct DOM manipulation with jQuery in some of my Vue.js sites.
I have to admit, the sites did not blow up as you would expect them to after being cautioned by some purists.
Nonetheless, it's not a good habit, and potentially creates performance, overhead, and debugging issues that we can easily avoid.
Instead, there are many libraries and packages out there that will offer you slideUp / slideDown-like behavior, and that suit whichever framework or vanilla environment you're working with. In this article I'm going to go over a few options you can reach for.
Vue 2
Despite Vue 3 being the default version now, many out there are still using the last major version. Nuxt.js's stable release is still using Vue2 and possibly other Vue variants as well.
For Vue 2, we have the @ztrehagem/vue-accordion NPM package. Customization is not really documented as far as I can tell, but the basic functionality is quite easy to set up.
Install
npm install @ztrehagem/vue-accordion
Usage
Then, you can use it inside a component as shown in this simple implementation
<template>
<div>
<button @click="isExpanded = !isExpanded">Toggle</button>
<VueAccordion :expanded="isExpanded">
<strong>Hello World!</strong>
</VueAccordion>
</div>
</template>
<script>
import VueAccordion from '@ztrehagem/vue-accordion'
import '@ztrehagem/vue-accordion/dist/vue-accordion.css'
export default {
data() {
return {
isExpanded: false,
}
},
components: {
VueAccordion,
}
}
</script>
Demo
https://stackblitz.com/edit/vue2-vue-cli-7tieme?file=src/components/HelloWorld.vue
Vue 3
For Vue 3 I demo'd vue-collapse-transition which I understand works with both Vue 2 and Vue 3.
Vue-collapse-transition offers a little more on configuration with things like timing functions and duration that can be modified via attributes.
Install
npm i @ivanv/vue-collapse-transition
Usage
Simple usage could look something like this
<template>
<div>
<button @click="isOpen = !isOpen">Toggle</button>
<collapse-transition>
<div v-show="isOpen">This div will open and close smoothly!</div>
</collapse-transition>
</div>
</template>
<script>
// Dist version is messed up. Need to import the component directly
import CollapseTransition from '@ivanv/vue-collapse-transition/src/CollapseTransition.vue';
export default {
components: {
CollapseTransition,
},
data() {
return {
isOpen: false, // closed by default
};
},
};
</script>
It's important to note that in Vue 3 you need to import the CollapseTransition.vue component directly as shown above.
Demo
https://stackblitz.com/edit/vitejs-vite-uaghgt?file=src/App.vue
React 17
For React, I found the react-collapse component. Unlike the two Vue plugins I mentioned above. react-collapse's documentation is much more technical with a lot of configuration explained as you'll see if you follow this link.
Install
npm i react-collapse
Usage
A very simple implementation could look something like this
import React, { useState } from 'react';
import './style.css';
import { Collapse } from 'react-collapse';
export default function App() {
const [isOpen, setIsOpen] = useState(false);
return (
<div>
<button onClick={() => setIsOpen(!isOpen)}>Toggle</button>
<Collapse isOpened={isOpen}>
Lorem ipsum dolor sit, amet consectetur adipisicing elit. Voluptate sit
architecto provident iure quos error eius natus harum porro laborum
deserunt, laudantium adipisci ex voluptatum maiores, sed numquam
quisquam. Minus, hic ipsa natus dolor aspernatur adipisci modi aut
placeat accusamus, quibusdam soluta porro alias? Facilis totam
recusandae molestiae aperiam ad!
</Collapse>
</div>
);
}
and you'll need to add some CSS to define the duration of the transition like this
.ReactCollapse--collapse {
transition: height 500ms;
}
Demo
https://stackblitz.com/edit/react-cu9vdl?file=src/App.js
Vanilla Javascript
Inside a Vanilla Javascript project, I have used es6-slide-up-down several times in production and it has done me just fine.
Install
npm i es6-slide-up-down
Usage
In your markup you can do something like
<div id="app">
<button>Toggle</button>
<div class="slide-wrapper" style="display: none">
<p>
Lorem ipsum dolor sit, amet consectetur adipisicing elit. Fugit molestiae
asperiores temporibus a facere quis repellendus facilis tempora quia rerum
ad eveniet cupiditate veniam, doloremque voluptate eaque debitis id
laudantium omnis tempore ratione laborum numquam. Cupiditate sunt fugit
voluptate. Molestiae, similique obcaecati. Impedit voluptates iusto, vel
eligendi accusantium, iure nisi ullam pariatur beatae harum voluptatum
laborum cupiditate sapiente optio. Ipsa.
</p>
</div>
</div>
and in your Javascript entry file we'll use a little logic to toggle our sliding
// Import stylesheets
import './style.css';
import { slideUp, slideDown } from 'es6-slide-up-down';
let isOpen = false;
const toggleButton = document.querySelector('button');
const content = document.querySelector('.slide-wrapper');
toggleButton.addEventListener('click', () => {
if (isOpen) {
slideUp(content);
isOpen = false;
} else {
slideDown(content);
isOpen = true;
}
});
As with the Vue plugins, there isn't much configuration covered in the docs, but with some playing around and tweaking you should be able to get there.
Demo
https://stackblitz.com/edit/js-grbpkn?file=index.js
Honorable Mention
Last, but certainly not least, be sure to check out the Alpine.js Collapse Plugin especially if you're already using Alpine.js in your applications. It does what the above packages do in a very simple and elegant way.
Conclusion
I hope you found any one, some, or all of these useful. Please share any useful nuggets in the comments below and helper others who may have also found there way here!
Code on web assassins.